Effective Development Using the pos-cli
When developing an application with platformOS, you need to use various pos-cli
commands. Most commonly used are sync
, logs
, and gui serve
. Apart from pos-cli
you might use some kind of assets bundler, like webpack in watch mode. When writing JavaScript, you might want to run your test runner in watch mode. If you want to reload your browser on every asset change, because you are styling the website, then you need another tool, for example, livereload.
These are a lot of tools to run at the same time, each of them taking up one terminal window, which becomes cumbersome to manage. This article aims to make this issue a non-factor.
npm tasks
After some research, trials and educated guesses, we figured that packaging everything into npm tasks and running them concurrently is the way to go.
The final result looks like this, and was used to develop the Todo App with no issues.
"scripts": {
"clean": "rm -rf app/assets/*",
"dev-logs": "pos-cli logs dev",
"dev-sync": "pos-cli sync dev --livereload",
"dev-gui": "pos-cli gui serve dev",
"dev-watch": "npx webpack-cli -w",
"start": "npx concurrently npm:clean npm:dev-*"
}
Read more about npm scripts.
We are using webpack for assets bundling, pos-cli built-in livereload to refresh the browser on changes, and concurrently to run multiple tasks in one terminal.
Having all those tasks defined one by one allows you to run them one by one when you need it:
$ npm run clean
Note
Keep in mind that the command rm -rf
does not exist on Windows, so if you are using Windows, change that command, or use rimraf for cross-platform compability.
npx
npx
is a tool that allows you to run npm packages that expose executable files (binaries). If the package is not installed on your system (locally or globally), it will install it and then execute.
You can run any npm package, without knowing if it's installed or not, just by prefixing it with npx
. For example, we could rewrite the clean task to be cross platform like this:
$ npx rimraf app/assets/*
Read more about npx.
livereload
Livereload is broadcasting a "refresh" message when files that you defined are changed. In pos-cli
livereload is watching app
and modules
directories and allowed extensions.
To enable LiveReload server you need to add --livereload
(or -l
) flag to your sync command.
After you have your livereload server running, you need a client.
Install the extension and when you are on a page that you want to be refreshed when files change, click the livereload extension icon.
Chrome extension
Firefox extension
Look for an icon that looks similar to .
concurrently
Finally, when you have all your tasks prepared, you need to run them all at once in one terminal, to avoid remembering long commands and occupying bunch of terminal windows.
There is a lot of tools that can do that, including bash scripting, but we decided to use the npm package concurrently.
It has more options than we used in this article, for example coloring:
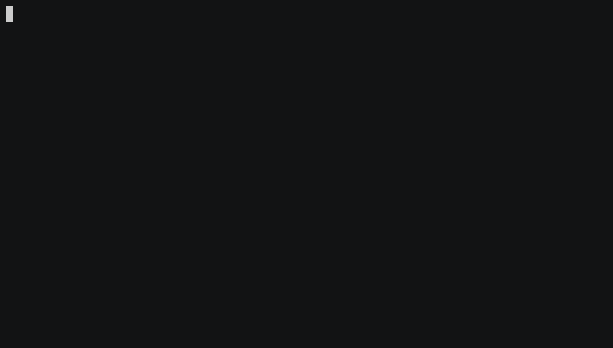
Putting it all together
Finally, when you have all atomic npm tasks doing one thing, it's time to join them together to run them all using one command.
"start": "npx concurrently npm:clean npm:dev-*"
Explanation:
- Use the
start
task because it is the most appropriate and you don't need to writerun
before it to run it. You just writenpm start
, which is convenient. - Use npx to run concurrently.
- Run the
clean
task to delete old assets. - Run all tasks where the name starts with
dev-
, in this case:
logs - runspos-cli logs dev
sync - runspos-cli sync dev
gui - runspos-cli gui serve dev
watch - runs webpack in development and watch mode
Final result
All of this in practice:
At the beginning, there will be a lot of text going through your screen, because every task needs to execute and all of them leave some kind of trace in the console. But after this initial wall of text, only observing tasks should output things to the terminal, such as sync, logs, and webpack.
All tasks run by concurrently stream their output to one terminal, and every line is prefixed with the name of the task that is responsible for a given entry.
It also shows the exit code if the task has finished, like it did with the clean task:
[clean] npm run clean exited with code 0
That way you have a trace when a task goes wrong.
That's it, this is the setup that can save you a lot of terminal windows, time and hopefully makes your development more efficient.